Explore SQLite JSON Operations Using JDBC
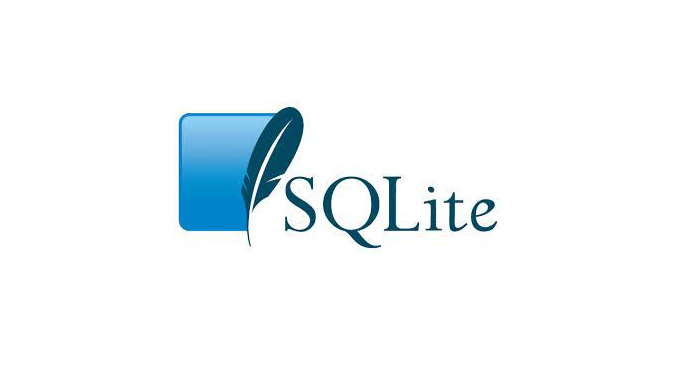
SQLite is an library that implements a self-contained, serverless, zero-configuration, transactional SQL database engine and provides support to create embedded database. SQLite is very well tested and most widely used in embedded platforms. It provides a complete SQL database with support for multiple tables, indices, triggers, and views, JSON Functions, Full text search and lot more.
In this article, we will explore SQLite JSON operations using JDBC. Refer to the article to learn about How to perform CRUD operations in SQLite using JDBC.
Storing JSON data in database is inevitable. Most of the database provide JSON functions to work with JSON column. We will explore few of the most important functions.
Dependency
Let's get started
Let's connect to the database and create statement
object.
Create table with JSON column.
Add few records to the employee
table.
Now we have added JSON data to the employee
table. Lets query and display the data.
Data will be displayed as below.
Now lets insert json field to existing JSON column. We will add countryCode to existing data column.
We can use either json_set
or json_insert
function. The function takes arguments like source json field, json path and value. Json path has to be refered as $.fieldname
.
Print the results.
We can remove a particular field from JSON column using json_remove
function. The functions takes source field and a json path as argument.
Extract a particular field from JSON field we can use ->
, ->>
operator or json_extract
function.
Below statement uses ->>
operator to access json field.
Below statement uses json_extract
function.
Conclusion
Using JSON fields are inevitable in database. SQLite database has very good performance in using JSON fields.