Basics of Dart for Web Development
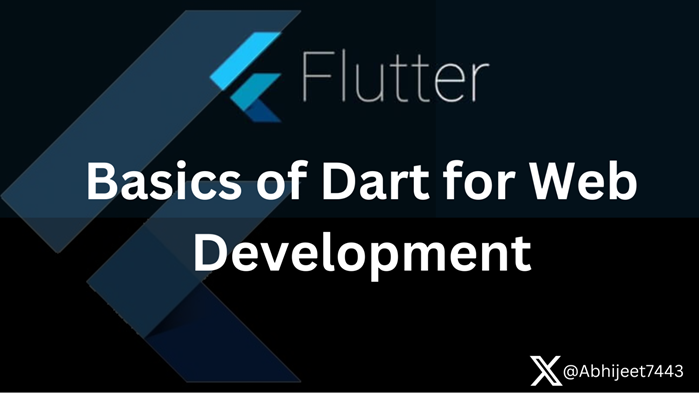
Dart is a programming language developed by Google, primarily for building web, mobile, and desktop applications. When it comes to web development, Dart offers a comprehensive set of features and tools to create modern, efficient, and scalable web applications. Here are some key basics of Dart for web development:
Syntax and Structure
Dart syntax is similar to many other languages like JavaScript, Java, and C#, making it relatively easy to learn for developers familiar with those languages. Dart code is structured into classes, functions, variables, and expressions, allowing for organized and readable code.
Strong Typing
Dart is a statically typed language, which means that variables have types that are known at compile time. This helps catch errors early in the development process and improves code reliability and maintainability.
Asynchronous Programming
Dart provides built-in support for asynchronous programming through features like async/await and Futures. This allows developers to write non-blocking code for tasks such as fetching data from APIs or performing I/O operations without blocking the main thread.
Package Management
Dart comes with its own package manager called Pub, which allows developers to easily manage dependencies and share their own packages with the community. Pub hosts thousands of packages for various purposes, including web development frameworks, utility libraries, and integration with other services.
Web Development Frameworks
Dart offers several web development frameworks to choose from, each with its own strengths and focus areas. Some popular frameworks include:
AngularDart
AngularDart is a framework for building complex web applications using Dart and Angular. It provides a rich set of features for building single-page applications (SPAs) with components, routing, and dependency injection.
Flutter for Web
While originally designed for mobile app development, Flutter also supports web development using the same Dart codebase. Flutter for Web enables developers to build beautiful and responsive web applications with a single codebase, leveraging Flutter's rich set of UI components and hot reload feature.
Aqueduct
Aqueduct is a server-side framework for building RESTful APIs and web servers in Dart. Aqueduct follows the MVC (Model-View-Controller) architecture and provides features like ORM (Object-Relational Mapping) for database interactions and authentication middleware.
Tooling and IDE Support
Dart developers have access to a range of tools and IDEs (Integrated Development Environments) for efficient development. The Dart SDK includes a command-line interface (CLI) for compiling, testing, and analyzing Dart code. Additionally, popular IDEs like Visual Studio Code and IntelliJ IDEA offer Dart plugins with features like code completion, debugging, and refactoring support.
Cross-Platform Development
With Dart, developers can build not only web applications but also mobile and desktop apps using frameworks like Flutter. This allows for code reuse across different platforms, saving time and effort in development and maintenance.
In summary, Dart provides a robust and versatile platform for web development, with features like strong typing, asynchronous programming, and powerful frameworks like AngularDart and Flutter for Web. With its growing ecosystem and community support, Dart continues to be a compelling choice for building modern web applications.
Server-side Dart Programming
Dart is a versatile language that can be used for both server-side and client-side programming, offering a unified approach to building web applications. Let's explore server-side and client-side Dart programming:
Dart VM and Server Applications
Dart provides a built-in Virtual Machine (VM) that allows developers to run Dart code on the server-side. This enables the creation of high-performance server applications using Dart.
Developers can build server-side applications, including web servers, RESTful APIs, and microservices, using frameworks like Aqueduct, which is specifically designed for Dart server development.
Frameworks and Libraries
Aqueduct: A server-side framework for Dart that follows the MVC architecture and provides features like ORM for database interaction, routing, authentication, and middleware support.
Shelf: A minimalist web server framework for Dart that allows developers to handle HTTP requests and responses with ease. It's flexible and can be used to build custom server applications tailored to specific requirements.
Database Connectivity
Dart provides libraries and packages for connecting to various databases, including SQL databases like PostgreSQL, MySQL, and SQLite, as well as NoSQL databases like MongoDB.
Libraries like `dart:io` and third-party packages such as `postgres`, `sqljocky`, and `mongodb` facilitate database interactions in Dart server-side applications.
Creating a Simple Server
You can create a basic HTTP server using Dart's built-in dart:io
library.
import 'dart:io';
void main() {
var server = HttpServer.bind(InternetAddress.loopbackIPv4, 8080);
print('Server running on localhost:${server.port}');
server.listen((HttpRequest request) {
request.response.write('Hello, Dart!');
request.response.close();
});
}
Client-side Dart Programming
Dart for Web
Dart can be compiled to JavaScript, allowing developers to use Dart for client-side web development.
With frameworks like AngularDart and Flutter for Web, developers can build interactive and responsive web applications using Dart.
Frameworks and Libraries
AngularDart: A web application framework that combines Dart with Angular, offering powerful features for building single-page applications (SPAs) with components, routing, and dependency injection.
Flutter for Web: Allows developers to build web applications using the same Dart codebase used for mobile app development with Flutter. It provides a rich set of UI components and hot reload for rapid development.
Dart HTML: Dart provides libraries for interacting with the HTML DOM (Document Object Model) and manipulating web page elements dynamically. These libraries simplify client-side development tasks like event handling, DOM manipulation, and AJAX requests.
Package Ecosystem
Dart's package manager, Pub, hosts numerous packages for web development, including libraries for HTTP requests, state management, form validation, and UI components.
Developers can leverage existing packages or publish their own packages to enhance the functionality of their client-side Dart applications.
Creating a Simple Client-side Application
You can use Dart to create interactive web applications by compiling Dart code to JavaScript.
import 'dart:html';
void main() {
querySelector('#output').text = 'Hello, Dart!';
}
Interacting with DOM
Dart provides the dart:html library for interacting with the DOM (Document Object Model) in web browsers.
import 'dart:html';
void main() {
var button = ButtonElement();
button.text = 'Click Me';
button.onClick.listen((event) {
querySelector('#output').text = 'Button Clicked!';
});
querySelector('#container').append(button);
}
Compiling Dart for Web
Dart code can be compiled to JavaScript for running in web browsers using tools like the Dart SDK or Dart Dev Compiler (dartdevc).
Example command to compile Dart to JavaScript:
dart compile js my_script.dart
By leveraging Dart for both server-side and client-side programming, developers can create full-stack web applications with a unified language and toolset, resulting in improved code consistency, productivity, and maintainability.
Dart offers a comprehensive ecosystem for web development, with frameworks and libraries for both server-side and client-side programming. Whether you're building RESTful APIs, web applications, or interactive user interfaces, Dart provides the tools and capabilities to create modern web experiences efficiently.
Example of Client-Side Dart Program
import 'package:flutter/material.dart';
import 'dart:html' as html;
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Click Me'),
),
body: Center(
child: ElevatedButton(
onPressed: () {
html.window.alert('Button Clicked!');
},
child: const Text('Click Me'),
),
),
),
);
}
}
Dart and RESTful APIs
Making HTTP requests in Dart is a fundamental aspect of building web applications, particularly when interacting with RESTful APIs. Dart provides several options for making HTTP requests, allowing developers to fetch data from external servers, send data to APIs, and perform various CRUD (Create, Read, Update, Delete) operations. Here's how you can make HTTP requests in Dart:
Using the `http` Package:
Dart's `http` package is a popular choice for making HTTP requests in Dart applications. It provides a simple and intuitive API for performing HTTP operations.
Installation
You can add the `http` package to your Dart project by adding it to your `pubspec.yaml` file:
dependencies:
http: ^0.13.3
Then, run pub get
or flutter pub get
to install the package.
Making GET Requests
import 'package:http/http.dart' as http;
void fetchData() async {
var url = Uri.parse('https://api.example.com/data');
var response = await http.get(url);
if (response.statusCode == 200) {
// Request successful, parse response data
var data = response.body;
print(data);
} else {
// Request failed, handle error
print('Failed to fetch data: ${response.statusCode}');
}
}
Making POST Requests
import 'package:http/http.dart' as http;
void sendData() async {
var url = Uri.parse('https://api.example.com/data');
var response = await http.post(url, body: {'key': 'value'});
if (response.statusCode == 200) {
// Request successful, handle response
print('Data sent successfully');
} else {
// Request failed, handle error
print('Failed to send data: ${response.statusCode}');
}
}
Handling JSON Data
import 'dart:convert';
import 'package:http/http.dart' as http;
void fetchJSONData() async {
var url = Uri.parse('https://api.example.com/data');
var response = await http.get(url);
if (response.statusCode == 200) {
// Parse JSON response data
var jsonData = json.decode(response.body);
print(jsonData);
} else {
// Request failed, handle error
print('Failed to fetch data: ${response.statusCode}');
}
}
Using Other Dart HTTP Client Libraries
In addition to the `http` package, Dart developers can also use other HTTP client libraries like `dio`, `http_io`, or `http_parser`, depending on specific project requirements and preferences. Each library offers its own set of features and capabilities for making HTTP requests in Dart applications.
By utilizing Dart's HTTP client libraries, developers can easily integrate RESTful APIs into their Dart applications, enabling seamless communication with external servers and services.
Conclusion
In conclusion, Dart provides powerful tools and libraries for making HTTP requests, facilitating seamless integration of RESTful APIs into Dart applications. By leveraging packages like `http`, developers can easily fetch data from external servers, send data to APIs, and handle JSON responses with minimal boilerplate code. Dart's asynchronous programming model ensures that HTTP requests can be made efficiently without blocking the main thread, enhancing the responsiveness and performance of web applications.
Furthermore, Dart's ecosystem offers a variety of HTTP client libraries, such as `dio`, `http_io`, and `http_parser`, providing developers with flexibility and choice based on project requirements and preferences. Whether building server-side applications, client-side web applications, or full-stack solutions, Dart empowers developers to interact with RESTful APIs effectively, enabling the creation of robust and scalable web applications.
With its intuitive syntax, strong typing, and comprehensive tooling, Dart simplifies the process of making HTTP requests, streamlining the development workflow and accelerating the delivery of web applications. As Dart continues to evolve, its capabilities for handling HTTP requests are expected to further improve, solidifying its position as a preferred language for web development.