Introduction To Play Framework
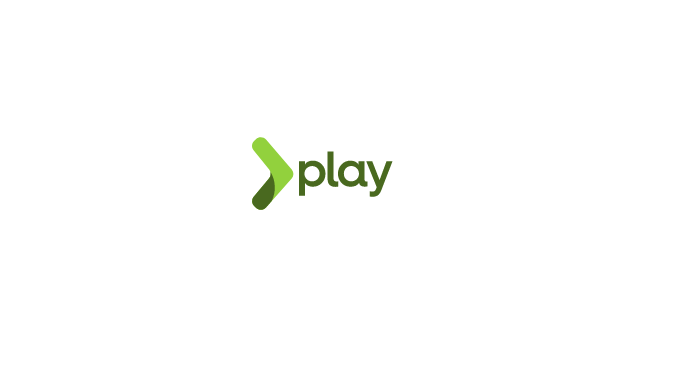
Play is a high-performance web application framework for JVM-based programming languages, like Java and Scala. We need it for developing modern web applications because it integrates the components and APIs we need.
Our goal in this tutorial is to explore Play Framework and learn how to build a web application.
What is Play framework?
With Play, you can develop high-quality Java and Scala web applications by integrating components and APIs. Play includes all the components you need for building Web Applications and REST services, including an integrated HTTP server, form handling, CSRF protection, powerful routing mechanisms, and I18N support.
Through hot reloading, Play simplifies everyday tasks and saves development time by directly supporting everyday tasks.
Under the hood, Play's lightweight, stateless, web-friendly architecture uses Akka and Akka Streams to consume CPU, memory, and threads in a predictable and minimal manner. Because of its reactive model, applications scale horizontally as well as vertically.
Features of the Java Play Framework
Modular architecture: Play Framework has a modular architecture, which makes it easy for developers to add and remove functionality as required. Framework components are prepackaged components that can be plugged into applications to add specific functionality. Developers can add modules for accessing databases, securing data, and more.
Reactive programming: With the Play Framework, you can use reactive programming, which emphasizes data processing asynchronously. Scalability, responsiveness, and resilience are all advantages of reactive programming.
Hot reloading: With Play Framework's hot reloading feature, developers can see their code changes in real time. As a result, the development process is sped up and the time it takes for changes to be seen is reduced.
Built-in testing: With Play Framework, you can test your application with built-in testing tools. As the framework supports both functional and unit tests, developers can test the entire application, from controllers to databases.
High performance: A key feature of the Play Framework is its speed and efficiency. With its stateless, non-blocking architecture, it can handle a large number of requests simultaneously. As a result, applications remain responsive and can scale as needed.
Easy deployment: Deploying an application is easy with Play Framework. Both standalone and clustered deployments are supported by the framework, so developers can choose the option that best suits their needs.
Benefits of Play Framework
Scalability and effectiveness: Play Framework's reactive structure allows it to handle concurrent requests effectively and produce high-performance applications that scale well.
Faster and more iterative development: With Play Framework's hot code reloading feature, developers can change their code without restarting the application.
Increased productivity: By simplifying development tasks and reducing boilerplate code, Play Framework simplifies development. Additionally, it has built-in testing tools, making designing and running tests easier and more productive.
Support for developing RESTful APIs: Play Framework simplifies the creation and maintenance of API-centric applications by offering a clear and expressive approach to designing and implementing web services. Developing RESTful APIs is easy with Play Framework.
Dependency injection: Play Framework supports dependency injection for modular development, which allows programmers to create applications that are loosely coupled and modular. Therefore, complicated projects can be maintained more efficiently and with better code organization and reuse.
The anatomy of play applications
Here, we'll learn what the files and directories in a Play application do and how they're structured.
Models: In this subcategory, you'll find business-related files that model database tables. Models, for instance, handle company tasks such as shipping and tax charges for websites for consumers.
Views: Here you'll find all browser-friendly HTML templates. The HTML in this subcategory is used for non-immediate purposes when loading a webpage. During productivity, HTML can be activated using JavaScript.
Controllers: Controllers are Java source files responsible for completing API requests or data inquiries between systems. The actions are defined as methods for processing and returning HTTP responses, which inform the user if a request has been successfully completed or has encountered an unexpected error.
Assets: This subcategory offers specialized functions based on your type of web development. As an example, if user experience is vital to the success of your business, your system may be configured to keep track of and analyze site statistics and improve customer satisfaction.
Environment setup:
In order to set up the stage for application development, the following steps must be completed. Here are the details of the configuration and installation.
In order to use this framework, you must first install Java (version 5 or higher) on your computer. Also, you can use any text editor or install an IDE.
Click here to download JDK and set the classpath
Install any IDE such as NetBeans or Eclipse
Next, download and install the Play framework.
You can download the Play framework (.zip file) from here following link. Unzip it to a local directory (for example, c:\Play).
Next, download the Scala
You can download and install Scala for Windows here. Follow the instructions onscreen to get started.
We will download the Play Java Hello World tutorial zip file and unzip it to a convenient location. The application can be run by running the sbt executable at the root of this folder.
Here's how to run the application
cd path_to_your_folder
sbt run
Upon running this command, we'll see a message that says "(Server started, press Enter to stop and return to the console...)".
Our application is now ready, so we can go to http://localhost:9000 and see the Play Welcome page:
We will create a very basic web application in this section. To familiarize ourselves with the Play framework, we will use this application.
Using the sbt new command, we can create a Play Framework application without downloading an example project.
We'll open a command prompt, navigate to our desired location, and run the following command:
sbt new playframework/play-java-seed.g8Copy
The above command will prompt us for a name for the project first. Next, it will ask for the domain (in reverse, as is the package naming convention in Java) that will be used for the packages. We press Enter without typing a name if we want to keep the defaults which are given in square brackets.
The application generated with this command has the same structure as the one generated earlier. We can, therefore, proceed to run the application as we did previously:
cd path_to_your_folder
sbt run
The above command, after completion of execution, will spawn a server on port number 9000 to expose our API, which we can access through http://localhost:9000. We should see the message “Welcome to Play” in the browser.
Our new API has two endpoints that we can now try out in turn from the browser. The first one – which we have just loaded – is the root endpoint, which loads an index page with the “Welcome to Play!” message.
In the second case, http://localhost:9000/assets, you can download files from the server by adding their names to the path. You can test this endpoint by getting the favicon.png file from http://localhost:9000/assets/images/favicon.png, which was downloaded with the application.
How to add Actions and Controllers
Actions are Java methods within a controller class that process parameters and send results to the client.
Controllers are Java classes that extend play.mvc.Controller, logically grouping actions according to the results they produce.
Now let's explore app-parent-dir/app/controllers and look at HomeController.java.
In the HomeController's index action, a simple welcome message appears:
public Result index() {
return ok(views.html.index.render());
}
In the views package, this is the default index template:
@main("Welcome to Play") {
<h1>Welcome to Play!</h1>
}
The index page calls the main template, as shown above. The main template then renders the page header and body tags. This function takes two arguments: a String for the title of the page and an HTML object for the body.
@(title: String)(content: Html)
<!DOCTYPE html>
<html lang="en">
<head>
@* Here's where we render the page title `String`. *@
<title>@title</title>
<link rel="stylesheet" media="screen" href="@routes.Assets.versioned("stylesheets/main.css")">
<link rel="shortcut icon" type="image/png" href="@routes.Assets.versioned("images/favicon.png")">
</head>
<body>
@* And here's where we render the `Html` object containing
the page content. @
@content
<script src="@routes.Assets.versioned("javascripts/main.js")" type="text/javascript"></script>
</body>
</html>
In the index file, let's change the text a bit:
@() @main("Welcome to Blackslate!") {
<h1>Welcome to Play Framework on Blackslate!</h1>
}
We will see a bold heading after reloading the browser:
If we remove the render directive from the index() method of the HomeController, we can return plain text or HTML text directly without using a template:
public Result index() {
return ok("REST API with Play by Blackslate");
}
After editing the code, we'll only see the text in the browser. There will be no HTML or styling here, just plain text:
If we wrap the text in header tags and pass it to Html.apply, we can output HTML just as easily. Play around with it if you like.
Let’s add a /blackslate/greeting
endpoint in routes:
GET /blackslate/greeting controllers.HomeController.greeting
Let's create the controller for this endpoint:
public Result greeting() {
return ok(Html.apply("<h1>Hello Everyone!!</h1>"));
}
We'll see the above text formatted in HTML at http://localhost:9000/blackslate/greeting
By customizing the response type, we have manipulated our response. In a later section, we'll explore this feature in greater detail.
Play Framework also has two other important features.
-
We compile our code changes on the fly, so reloading the browser reflects the most recent version of our code.
-
The play.mvc.Results class provides helper methods for standard HTTP responses. We pass a response body as a parameter to the ok() method, and it returns an OK HTTP 200 response. The method for displaying text in the browser has already been used. In the Results class, there are more helper methods such as notFound() and badRequest().
How to manipulate results
We have been utilizing Play's content negotiation feature without even realizing it. Play automatically determines the response content type from the response body. We have been able to return the text in the ok method because of this:
return ok("Hello!!");
Play would then set the Content-Type header to text/plain. Although this works in most cases, we can customize the header by taking control.
To customize the response for the HomeController.customContentType action, let's use text/html:
public Result customContentType() {
return ok("This text content is present in the form of").as("text/html");
}
This pattern applies to all types of content. Text/html can be replaced with text/plain or application/json depending on the format of the data we pass to the ok helper method.
Conclusion
A flexible framework for online development, Play Framework prioritizes developer productivity and scalability. Due to its reactive and non-blocking design, it is well-suited for real-time and event-driven applications.
Backend development is easier with Play's support for RESTful APIs, data persistence, routing, and JSON serialization. With Play Framework, you can develop cutting-edge, effective, and responsive online applications.