Mastering API Integration in React Native with Expo: A Comprehensive Guide
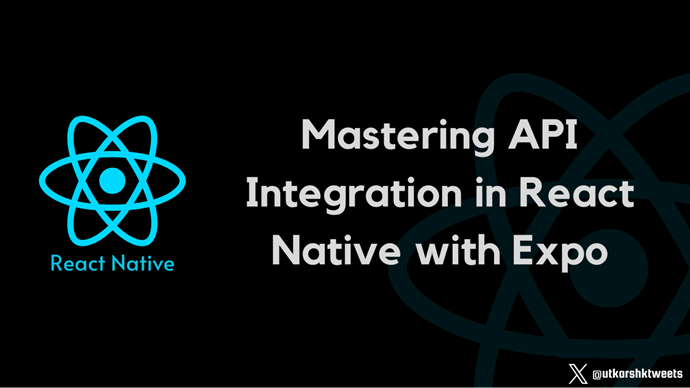
Welcome to the master class on API integration in React Native using Expo! In this extensive guide, we'll delve deep into the world of APIs (Application Programming Interfaces), exploring their significance, understanding how to make API calls in React Native, and mastering the art of handling API responses. Along the way, we'll build a News Reader App to practically apply the concepts covered. Let's embark on this journey to become API integration experts!
To learn basics of React Native and Expo, refer to our react native series post.
Understanding APIs: A Foundation for Mobile Development
What is an API?
An API, or Application Programming Interface, is a set of rules and protocols that allows one software application to interact with another. In the context of mobile development, APIs act as bridges, enabling mobile apps to communicate with external services, retrieve data, and perform various operations.
Why are APIs Essential?
APIs are the backbone of modern mobile development for several reasons:
1. Data Retrieval: APIs allow apps to fetch data from external sources, providing real-time and dynamic content.
2. Service Integration: They enable integration with third-party services, unlocking a plethora of functionalities without reinventing the wheel.
3. Scalability: APIs facilitate modular development, making it easier to scale and extend the functionality of an app.
4. Cross-Platform Compatibility: APIs enable developers to build cross-platform apps that can run on both iOS and Android.
Types of APIs
1. Web APIs (HTTP-based): These APIs use standard web protocols (HTTP/HTTPS) for communication. RESTful and GraphQL APIs fall into this category.
2. Library-Based APIs: Some APIs are packaged as libraries or SDKs (Software Development Kits), providing specific functionalities.
3. Hardware APIs: These APIs interact with device-specific features, such as camera APIs or sensor APIs.
Making API Calls in React Native
Setting Up Your Project
Let's start by creating a new React Native project using Expo. Open your terminal and run the following commands:
expo init NewsReaderApp
cd NewsReaderApp
Installing Dependencies
For making API calls, we'll use the Axios library. Install it using the following command:
expo install axios
Building a News Reader App: A Practical Application
File: App.js
import React, { useState, useEffect } from "react";
import {
View,
Text,
FlatList,
TouchableOpacity,
StyleSheet,
} from "react-native";
import axios from "axios";
const NewsReaderApp = () => {
const [news, setNews] = useState([]);
useEffect(() => {
const fetchNews = async () => {
try {
const response = await axios.get(
"https://newsapi.org/v2/top-headlines",
{
params: {
country: "us",
apiKey: "YOUR_API_KEY",
},
}
);
setNews(response.data.articles);
} catch (error) {
console.error("Error fetching news:", error.message);
}
};
fetchNews();
}, []);
const renderNewsItem = ({ item }) => (
<TouchableOpacity style={styles.newsItem}>
<Text style={styles.newsTitle}>{item.title}</Text>
</TouchableOpacity>
);
return (
<View style={styles.container}>
<View style={styles.header}>
<Text style={styles.logoText}>News Reader</Text>
</View>
<FlatList
data={news}
keyExtractor={(item) => item.url}
renderItem={renderNewsItem}
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
padding: 16,
backgroundColor: "#f0f0f0",
marginTop: 32, // Added margin to avoid overlapping with the front camera
},
header: {
alignItems: "center",
marginBottom: 16,
},
logoText: {
fontSize: 24,
fontWeight: "bold",
},
newsItem: {
backgroundColor: "white",
borderRadius: 8,
padding: 16,
marginBottom: 16,
},
newsTitle: {
fontSize: 18,
fontWeight: "bold",
},
});
export default NewsReaderApp;
NOTE: Go to https://newsapi.org/ and sign-up to get an API key and then replace YOUR_API_KEY with it.
Now, let’s understand each and every subpart of our code:
Writing Your API Call
Now, let's see how an API call is written and how it works :
import React, { useState, useEffect } from 'react';
import { View, Text, FlatList, TouchableOpacity, StyleSheet } from 'react-native';
import axios from 'axios';
const NewsReaderApp = () => {
const [news, setNews] = useState([]);
useEffect(() => {
const fetchNews = async () => {
try {
const response = await axios.get('https://newsapi.org/v2/top-headlines', {
params: {
country: 'us',
apiKey: 'YOUR_API_KEY', // Replace with your API key
},
});
setNews(response.data.articles);
} catch (error) {
console.error('Error fetching news:', error.message);
}
};
fetchNews();
}, []);
// ... (remaining code)
};
In this example, we use the Axios library to make a GET request to the News API, fetching top headlines from the United States. The useEffect
hook ensures that the API call is made when the component mounts.
Handling API Responses
API responses can be dynamic, and handling them effectively is crucial. Axios provides a convenient way to manage responses and errors. In our example, the response is stored in the news
state variable.
// ... (previous code)
useEffect(() => {
const fetchNews = async () => {
try {
const response = await axios.get('https://newsapi.org/v2/top-headlines', {
params: {
country: 'us',
apiKey:"YOUR_API_KEY", // Replace with your API key
},
});
setNews(response.data.articles);
} catch (error) {
console.error('Error fetching news:', error.message);
}
};
fetchNews();
}, []);
// ... (remaining code)
In case of a successful response, the setNews
function is called to update the news
state with the fetched articles. If an error occurs, it's caught in the catch block
, and an error message is logged.
Styling the App
Styling is an essential aspect of creating a user-friendly app. We've added styling to our app to provide a visually appealing and organized interface.
// ... (previous code)
const styles = StyleSheet.create({
container: {
flex: 1,
padding: 16,
backgroundColor: '#f0f0f0',
marginTop: 32,
},
header: {
alignItems: 'center',
marginBottom: 16,
},
logoText: {
fontSize: 24,
fontWeight: 'bold',
},
newsItem: {
backgroundColor: 'white',
borderRadius: 8,
padding: 16,
marginBottom: 16,
},
newsTitle: {
fontSize: 18,
fontWeight: 'bold',
},
});
// ... (remaining code)
In the styles
object, we've defined styles for the main container, header, logo text, and news items. These styles enhance the visual appeal and readability of our app.
Enhancing the User Interface
To provide a clear separation between the header and the list of news articles, we've added a View
component for the header, containing the logo text.
// ... (previous code)
return (
<View style={styles.container}>
<View style={styles.header}>
<Text style={styles.logoText}>News Reader</Text>
</View>
<FlatList
data={news}
keyExtractor={(item) => item.url}
renderItem={renderNewsItem}
/>
</View>
);
// ... (remaining code)
This enhancement improves the app's organization and user experience.
Conclusion
Congratulations! You've completed a masterclass on API integration in React Native using Expo. We covered the fundamentals of APIs, learned how to make API calls, and applied this knowledge to build a News Reader App. Understanding APIs is a cornerstone of mobile development, enabling you to create dynamic, data-driven applications.
As you continue your journey, consider exploring more advanced topics, such as authentication, handling different HTTP methods, and integrating with diverse APIs. Building on this foundation will empower you to create robust and feature-rich mobile applications.
Happy coding!